MOS, Summer 2009. A first VB program
MOS - Summer 2009
Below are instructions on how to create a simple Visual Basic program.
- Decide in advance what you want your program to look like and how you
want it to work.
- First, open the Visual Basic program. If you don't have an
icon for it on your desktop, then look for it under the "Start/Programs" menu.
- When you first open the program, it starts with the "New Project"
menu already open. For us, each project represents a program (an
application), and we will only do projects of type "Standard.EXE".
So just click "Open". If the "New Project" menu is not open and you
want to create a new project, then click on the "File/New Project" menu.
-
The Visual Basic program window has many parts. We'll give them names,
so we can refer to them easily.
Here's a screen shot of Visual Basic, with its parts labelled:
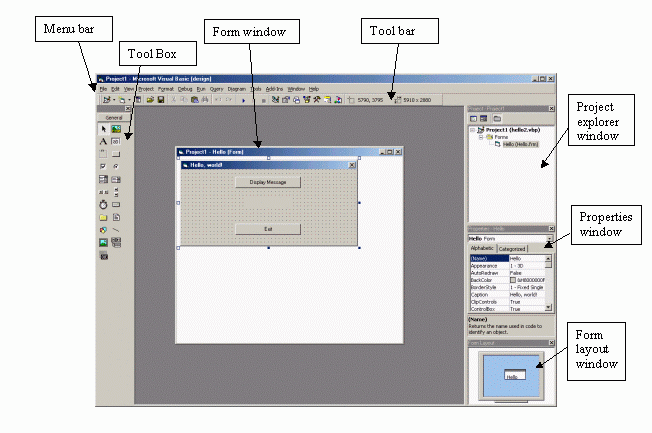
- When you start Visual Basic, you are automatically set up with an object
called Form1, and it is selected -
you can see what it looks like in the Form Window. It starts out empty.
Think of this form as the window that holds your program. Since the
form itself is selected (note the black squares on its corners and edges),
the Properties Window shows its list of properties. Change its name (preferably
to a name that starts with Form and reflects the purpose of your program).
Change its caption, which will show up as the title when you run the
program. You may also want to change MaxButton and/or MinButton if you want
to disallow maximizing or minimizing your application. Alternately, this
can be done by changing the BorderStyle to 1-Fixed Single.
- Resize the Form if you want to. You can also move its position in
the Form Layout window. This determines where the program window shows up
on the screen when you start the program.
- Find the Tool Box area of the VB window. The icons in the Tool Box
are for creating "controls". The purpose of controls is to give the user
a way of interacting with your program.
Position the mouse over one of the icons, and wait a moment - a "tooltip"
appears that tells you what that icon is for. Find the icon for
a Label, a TextBox, and a CommandBox. A Label is used for text that the
user cannot ever change, a TextBox is used for a user to type text into
the program, and a CommandBox is used for a user to click, usually
to tell the program to do something.
- Double-click on one of the control icons (whichever one you think you
need). A control appears in your Form. Move it and resize
it if you need to. Add whatever other controls you need, until your form
looks exactly like you want the program to look. If you click the
"Format" menu in the menu bar, there are selections there that can help you
align your controls.
- For each control, you need to make some changes to the Properties.
Select one of the controls, and look at the Properties Window.
- For labels,
change the Name so it starts with lbl and reflects the purpose of the label.
Change the caption to whatever you want the label to say. You may choose
to set the AutoSize property to "True", if you want the size of the label
to be based on the length of your caption. If you want to, experiment
with changing BackColor, BackStyle, BorderStyle, Font, ForeColor. If you
change ToolTipText, it describes the control for the user when they move
the mouse over it.
- For CommandButtons, change the Name so it starts with cmd and
reflects the purpose of the button. The Caption property gives the
text that the user will see on the button. Experiment with other properties
if you want to.
- For TextBoxes, change the Name so it starts with txt and reflects
the purpose of the text box. The Text property gives the default text -
this is the text that shows up in the box automatically for the user.
In many cases it makes sense to leave it blank.
It may seem strange to you that these controls have two identifiers - a
Name as well as a Caption or Text. The Name is what we call the control
internal to the program, while the caption or text is what the user sees.
- At this point, you can actually do a test run of the program.
Go to the menu bar
and click "Run/Start". You'll see your program appear on the screen just
as it will for the user, but it will be on top of the VB environment.
You can type into any TextBoxes, but when you try clicking on the buttons,
nothing happens. This is because you haven't yet written the code that
says what to do when these buttons are pushed.
- Now is a great time to save your program. Click on
"File/Save Project As". You'll notice that it will ask you to give two
different file names. One of these files ends with ".frm", and contains
the information for the form itself, and all the controls. The second
file ends with ".vpb" and contains the actual code. You need to save both
files.
- Now it's time to write the actual code itself. VB is an "event-driven"
programming environment. This means that the code is broken up into pieces,
each of which gets activated depending on what the user does. The user
can trigger these events by clicking on buttons, typing, moving the mouse,
etc. When you designed the user interface and the algorithmn, you should have
decided what tasks you want to perform,
and how you want the user to make those tasks happen. The task you
want to perform dictates what goes in the code. How you intend the user
to trigger those tasks determines where that code goes. You need to
decide both which control is involved (a TextBox, a CommandButton, etc.),
and what the user should do to that object to make the event happen.
- Double click on the control you want to attach code to. A code window
should show up. At the top left of this window you'll see the name of
the control, and at the top right is the name of the action. Your first
few programs will deal mainly with CommandBoxes and Clicks. But there are
many other options.
- Notice that a couple lines of code have automatically been written for
you, and there's a blank space in-between. This blank space is where you
insert the code (the program) for that event. For your first program,
you just need to know how to do three things: how to exit the
program
(the "End" command), how to pop up a message box (the "MsgBox" command), and
how to put a command into your code. You can find examples of all of these
in Simple sample programs.
- When you're done writing the code, try running the program again.
(Select "Run/Start" from the menu bar). The buttons in your program
are now "hooked-up" - when they're clicked, the code you wrote is executed.
To end the program, click the "X" icon, or select "Run/End" from the
menu bar.
- Test your program thoroughly, and fix any errors.
- When you're done with your program, you'd like to be able to run it
directly from Windows, without having to run Visual Basic. To do this, click
on "File/Make YourProgramName.exe". You'll save the executable to
somewhere in the file system. It is now pretty much a stand-alone program.
Open it from Windows, and your program runs.